This is a quite simple and strait forward task. Firstly add the compiler path to system path.:C: Program Files Microsoft Visual Studio 10.0 VC bin; Next, open command prompt and change directory to your source folder; Then execute the vcvars32.bat file to setup the environment for using vc on x86; After this, you can now type cl to compile your program. Other date stuff from cl wrksysval.DATTIM All my answers were extracted from the 'Big Dummy's Guide to the As400' and I take no responsibility for any of them. Code400 Newbie. Join Date: May 2005; Posts: 39; Share Tweet #3. February 9th, 2006, 09:41 AM. You can use the following code to get the current system date and time in C. Program output: The current time is 11:43:41 am The current date is 6-18-2015 June Wednesday Day of month is 17 and the Month of year is 6, also the day of. Alas, in CL an.ISO date must contain its date separators, YYYY-MM-DD. Fortunately there is a similar date format.YYMD which will give me what I desire without date separators. Lines 12 and 13: I am converting the character values in &FROMDATE and &TODATE from.MDY to.YYMD using the Convert Date command, CVTDAT.
- How To Get System Date In Cl Programs
- How To Retrieve System Date In Cl Program
- How To Get System Date In Cl Program Tutorial
January 19, 2005 Ted Holt
The code for this article is available for download.
A common saying is, 'It's the little things that get you.' The maxim was certainly true recently in one iSeries shop. I may not have all the details exactly right, but it seems nobody was able to pry any information out of the computer. Investigation revealed an unanswered message to QSYSOPR and a queue of jobs eagerly waiting for a chance to run. Apparently, the unanswered message owed its existence to the fact that some human being had entered an invalid date value into a prompt screen.
My impression is that this problem arose because of a combination of sloppy programming and CL's inadequate date-handling abilities (mostly the former). This problem could have been avoided. The program that accepted the user's input could have validated the date. But even that might have not been sufficient. If the user had keyed a valid, but unreasonable, date, there might still have been a problem.
In the following paragraphs I am going to provide you with some routines that can turn CL into a decent date-handler. As for the sloppy programming, that part's up to you.
Minimal CL Date-Handling
CL has not won any awards for its date-handling ability. Here is a complete list of the CL commands that manage dates.
1. CVTDAT
That's not much of a list, huh? The Convert Date (CVTDAT) command was designed to convert dates from one format to another. Since it chokes on invalid dates, it can also be used to verify that a variable contains a valid date value.
Here's a typical example that uses minimal CL to validate dates from a prompt screen. When the user requests a report, the system prompts for a range of dates.
Here's the DDS for the display file. Besides the prompt format, there is a message subfile for error messages.
Here's the code for an OPM CL program.
For this program to run correctly, you'll need a message description.
That doesn't look so hard, does it? The CVTDAT commands will generate an escape message, such as CPF05555 (Date not in specified format or date not valid) and CPF0557 (Date too short for specified format) if the user keys an invalid date. The global Monitor Message (MONMSG) command kicks in and branches to the Error routine, which sends the message to the program message queue, from which the display file retrieves and displays it to the user. One would think that any CL programmer could at least do this much to verify the accuracy of a date.
This example also includes one check for reasonableness: the first date in the range must not be after the second date in the range.
Once the user has entered valid dates, this program submits another program to batch, passing along the dates as parameters. Since it's possible that this program could run from somewhere else, such as the job scheduler, the submitted program should not assume that the dates are okay, but should perform validation of its own. If a date is found to be invalid, or possibly unreasonable, the program can send an escape message to cancel itself.
Had the programmer, who wrote the CL program about which I spoke in the introduction, included such a check, the job queue would not have filled up with requests.
Robust Date-Handling
In many situations a minimal amount of verification may be sufficient, but in other situations you may need something more robust. For example, December 25, 1969, is a valid date, but it may not make sense in some contexts. If you ask a user to enter an ending date for sales history inquiry, does it make sense to allow him to enter some date in the future?
If you really want robust date validation, you'll have to do what I've done, and come up with something of your own. I wrote an RPG module, CLDATERTNS, of date-handling subprocedures designed with CL in mind. You're free to use it and enhance it. The following table lists the subprocedures that I have chosen to include so far. Here's the source code for the CLDATERTNS member. The comments at the beginning will tell you how to create the module.
Subprocedure | Arguments | Return | Description |
AddDays | Date, | Date | Add |
AddMonths | Date, | Date | Add |
AddYears | Date, | Date | Add |
CurrDate | Date | Job date | |
CurrMonthBegin | Date | First | |
CurrMonthEnd | Date | Last date | |
DaysDiff | Date, | Number | Number of |
IsNotValidDate | Date | Logical | True if |
IsValidDate | Date | Logical | True if |
MonthBegin | Date | Date | First |
MonthEnd | Date | Date | Last date |
PrevMonthBegin | Date | First | |
PrevMonthEnd | Date | Last date |
The dates used by these routines are six-byte character values in job date format. Although I use them with *MDY dates, I have briefly tested with other date formats and the routines appear to work correctly. Numbers are five-digit packed decimal values with no decimal positions. Logical values are one-byte each, with 0 and 1 meaning false and true, respectively.
All routines except IsNotValidDate and IsValidDate send escape message USR2101 if you pass them an invalid date. The AddDays, AddMonths, and AddYears routines send escape message USR2102 if you pass them an invalid packed decimal argument. Here are the commands to create the message file and the messages. Feel free to rename the messages or put them in another message file.
Let's take the same application but make it more robust. I've made a slight change to the display file; I've added attributes to display invalid date fields in reverse image.
The CL program has changed considerably. The biggest change is that I've replaced one OPM CL program with an ILE program built from a CL module and the CLDATERTNS module. As I wrote in 'Optional Parameters and CL Procedures,' there are good reasons to dump OPM CL. This application provides yet another reason.
The revised CL uses the same basic logic, but the CVTDAT commands are gone, replaced with calls to procedures from CLDATERTNS. Rather than let the global MONMSG pick up exceptions, I've taken control of the validation process. This lets me check both dates for validity in one pass; whereas the minimal version stops looking for errors when a date proves invalid. Since I'm not passing along the system escape messages, I need a message of my own.
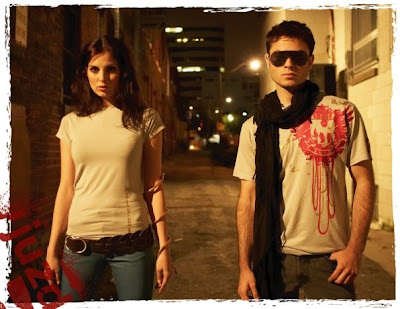
I've added one additional test. I've added code to verify that the dates are not more than 60 days in the past or 30 days in the future. The error message for this test requires that message JKL1002 be defined in the JKLMSG message file.
To create the program requires that I first create the two modules, and then bind them together to form a program. The instructions to create module CLDATERTNS are in the source code. Here are the remaining steps.
For Want of a Valid Date
I've concentrated on working with dates, but the lesson we should learn applies to all types of data. Never assume anything is correct. The old rhyme says that a kingdom was lost for want of a nail. In this case, money may have been lost for want of a valid date. Even if no money was lost, the inconvenience caused to users couldn't have had positive consequences.
Combine these date-handling routines with Cletus' short-cut date entry technique, and you may be on to something. But that's a subject for another day.
Click here to contact Ted Holt by e-mail.
The default history command output is always sorted by date unless there are any changes made to the configuration. The output is not in date order because session writes their history at different times. History file is written when the session gets over. Follow the steps given below to the date of execution in the history command output.
The default output of history command is as shown below without the date and time details:
Getting date and time of executed command in the history command output
How To Get System Date In Cl Programs
How To Retrieve System Date In Cl Program
1. Edit file /etc/bashrc and add the below entry. Make sure to have the space after %T.
2. Login in to the system.
3. Check if variable has been exported.
4. Type history command and check if time is getting displayed.
How To Get System Date In Cl Program Tutorial
5. To have output sorted according to date run on use the sort command along with the history command.
CentOS / RHEL : How to disable BASH shell history
UNIX / Linux : Examples of bash history command to repeat last commands